Member-only story
New in SwiftUI 3: SwiftUI 3 Animation in iOS 15
Like everything else in SwiftUI, attaching animation to your view is as easy as eating cake 😋. SwiftUI 3 brings more control over how animations are applied to the views.
New syntax:
@inlinable public func animation<V>(_ animation: Animation?, value: V) -> some View where V : Equatable
Omitting value
parameter in SwiftUI doesn’t throw error yet but you get deprecation warning:
'animation' was deprecated in iOS 15.0: Use withAnimation or animation(_:value:) instead.
animation function without value
parameter is marked to be deprecated in API:
@available(iOS, introduced: 13.0, deprecated: 15.0, message: "Use withAnimation or animation(_:value:) instead.")@inlinable public func animation(_ animation: Animation?) -> some View
So what’s will this value parameter do? 🤨
Value parameter provides a way to control when the animation should be played. Consider code below:
struct AnimationChanges: View {
@State private var offset: CGFloat = 0
@State private var scaleValue: CGFloat = 1
var body: some View {
VStack {
Text("DevTechie")
.font(.largeTitle)
.offset(x: offset)
.scaleEffect(scaleValue)
.animation(.easeInOut(duration: 5))
Spacer()
HStack {
Spacer()
Button("Change Offset") {
offset += 100
}
Spacer()
Button("Change Scale") {
scaleValue += 1
}
Spacer()
}
}
}
}
Pay attention to animation
modifier. We are telling the code that play the animation over 5 seconds. When you change scaleValue
or offset
value the animation will play.
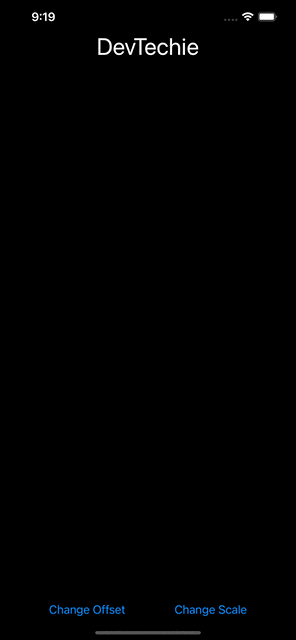
What if you only want to animate when value of scale changes but not when offset changes? This is when new value
parameter comes into play. Change your code like below:
struct AnimationChanges: View {…